test blog post with a name that is much too long
2025 Mar 11
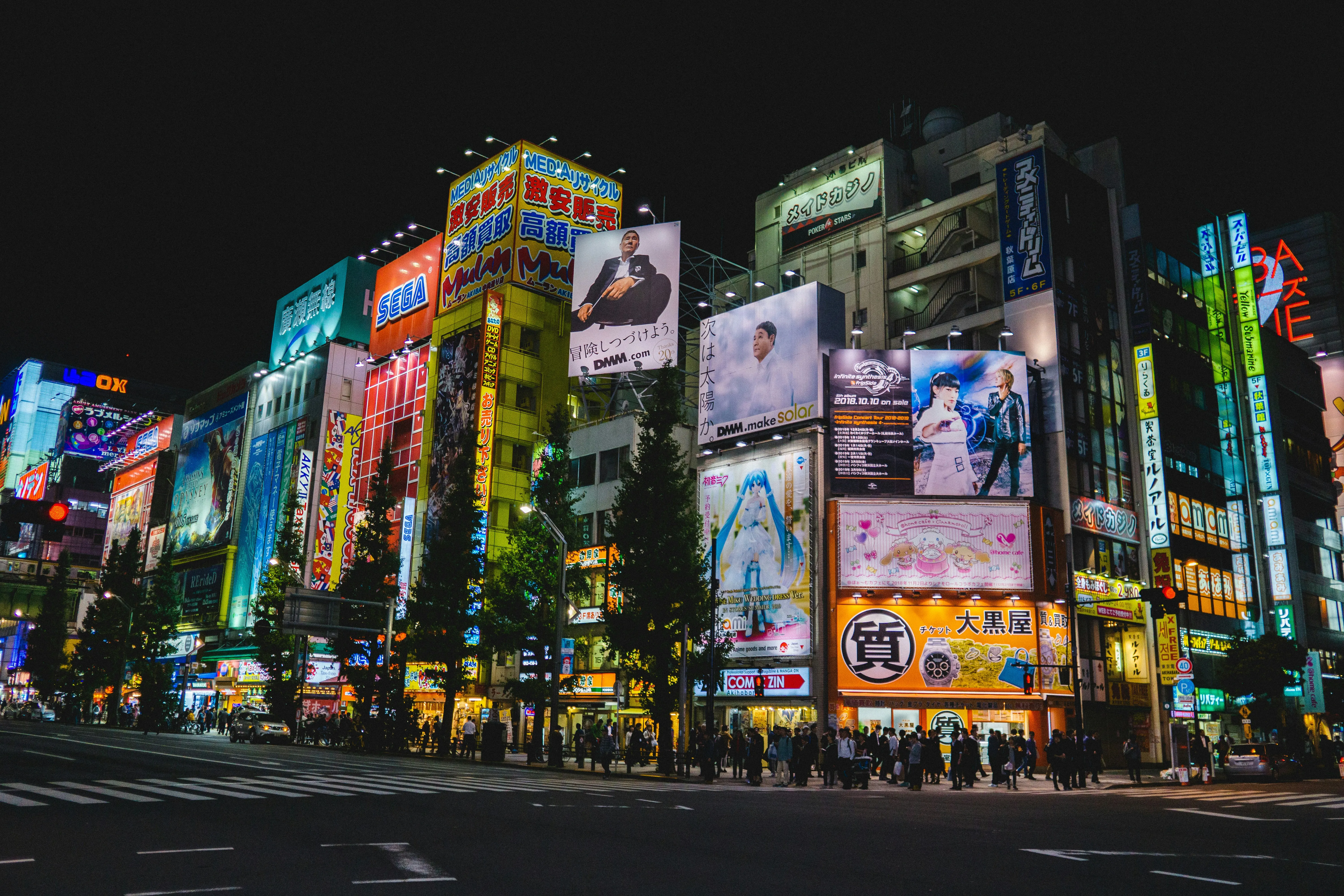
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Duis nibh elit, commodo vel nulla eu, pharetra aliquam justo. In malesuada dignissim risus eu facilisis. Donec elementum dui condimentum, interdum risus sed, cursus felis. Aenean et nisi diam. Sed hendrerit commodo magna, in tempus enim pretium quis. Vivamus vehicula ante vitae imperdiet faucibus. Vivamus suscipit varius aliquam. Nam pharetra semper lectus, interdum tempus magna scelerisque nec. Cras eget efficitur justo. Mauris lobortis dolor ac ante ultricies, vitae sollicitudin orci auctor. Quisque maximus semper tempus. Vestibulum vitae fringilla lacus. Aenean faucibus ex vitae purus iaculis, at varius erat ullamcorper. Nullam massa lectus, condimentum sed lacus id, faucibus faucibus ex. Nulla egestas, massa eget mollis pellentesque, urna elit mattis ante, non elementum felis felis vel orci.
Heading 1
Heading 2
Heading 3
Heading 4
Heading 5
Heading 6
italicized text bold text
- list item
- list item
- list item
- number
- number
- number
Did you hear that? They’ve shut down the main reactor. We’ll be destroyed for sure. This is madness! We’re doomed! There’ll be no escape for the Princess this time. What’s that? Artoo! Artoo-Detoo, where are you? At last! Where have you been? They’re heading in this direction.
I’m afraid she’s scheduled to be terminated. Oh, no! We’ve got to do something. What are you talking about? The droid belongs to her. She’s the one in the message.. We’ve got to help her. Now, look, don’t get any funny ideas.
Yeah, I think your right. Full reverse! Chewie, lock in the auxiliary power. Why are we still moving towards it? We’re caught in a tractor beam! It’s pulling us in! But there’s gotta be something you can do! There’s nothin’ I can do about it, kid. I’m in full power. I’m going to have to shut down. But they’re not going to get me without a fight! You can’t win.
You passed directly through a restricted system. Several transmissions were beamed to this ship by Rebel spies. I want to know what happened to the plans they sent you. I don’t know what you’re talking about.
This is Red Two. Flying toward you. Red Three, standing by. Red Leader, this is Base One.
Here’s an example of inline code
.
Here’s an example of a mark.
# import the required modules
import datetime
def gettime():
"""
A function to return the current time.
Returns
-------
tuple:
A tuple containing the hour, minutes and seconds.
"""
now = datetime.datetime.now()
return now.hour, now.minute, now.second + 1e-6 * now.microsecond
# get the time
hour, minute, seconds = gettime()
print(f"The current time is {hour}:{minute}:{seconds}")
# Everything after "#" is a comment.
# A file is a class!
# (optional) icon to show in the editor dialogs:
@icon("res://path/to/optional/icon.svg")
# (optional) class definition:
class_name MyClass
# Inheritance:
extends BaseClass
# Member variables.
var a = 5
var s = "Hello"
var arr = [1, 2, 3]
var dict = {"key": "value", 2: 3}
var other_dict = {key = "value", other_key = 2}
var typed_var: int
var inferred_type := "String"
# Constants.
const ANSWER = 42
const THE_NAME = "Charly"
# Enums.
enum {UNIT_NEUTRAL, UNIT_ENEMY, UNIT_ALLY}
enum Named {THING_1, THING_2, ANOTHER_THING = -1}
# Built-in vector types.
var v2 = Vector2(1, 2)
var v3 = Vector3(1, 2, 3)
# Functions.
func some_function(param1, param2, param3):
const local_const = 5
if param1 < local_const:
print(param1)
elif param2 > 5:
print(param2)
else:
print("Fail!")
for i in range(20):
print(i)
while param2 != 0:
param2 -= 1
match param3:
3:
print("param3 is 3!")
_:
print("param3 is not 3!")
var local_var = param1 + 3
return local_var
# Functions override functions with the same name on the base/super class.
# If you still want to call them, use "super":
func something(p1, p2):
super(p1, p2)
# It's also possible to call another function in the super class:
func other_something(p1, p2):
super.something(p1, p2)
# Inner class
class Something:
var a = 10
# Constructor
func _init():
print("Constructed!")
var lv = Something.new()
print(lv.a)